DPST1091/CPTG1391 Programming Fundamentals! - Assignment 2 - CS Pet Salon
CS Pet Salon
Overview
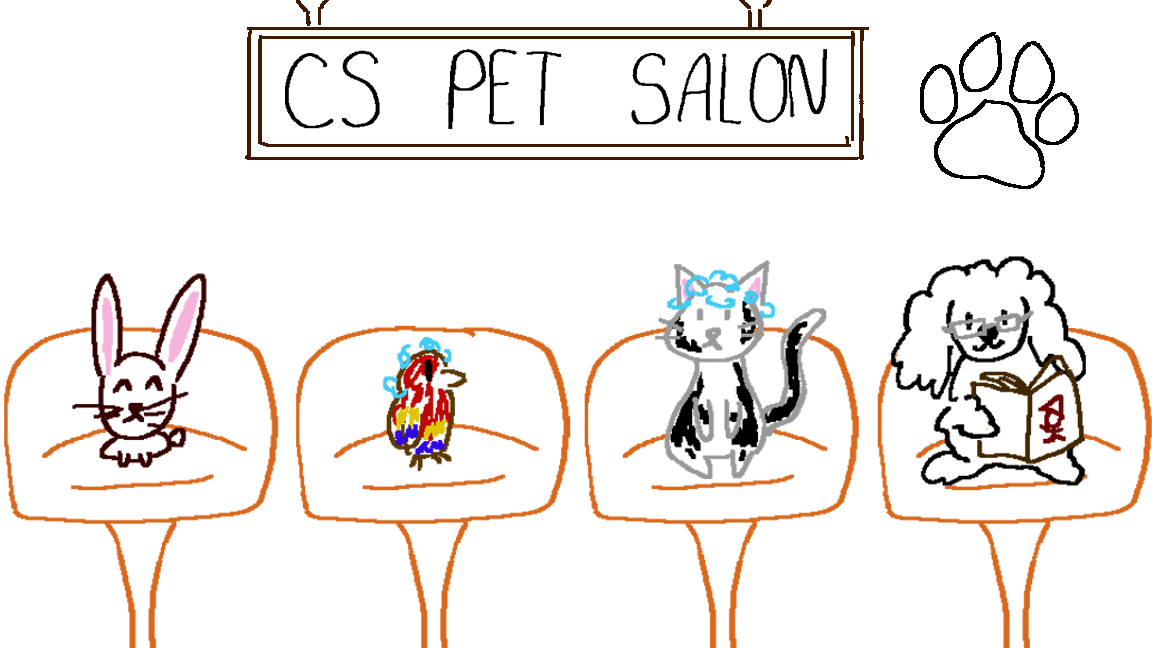
Assignment Structure
This assignment will test your ability to create, manipulate, and use linked lists to solve a variety of problems. To do this, you will be implementing a system to keep track of pet salons!
We have defined some structs in the provided code to get you started. You may modify any of the structs if you wish, but you should not need to.
struct salon
- Purpose:
- To store all the information of a salon
- Fields:
char salon_name[MAX_NAME_LEN]
- The name of the salon
struct financial_summary summary
- A struct for all the finances relating to the salon
double base_cost
- A double to keep track of the base cost of the salon
struct pet_room *rooms
- A pointer to the start of the list of pet rooms
struct salon *next
- A pointer to the next salon
struct pet_room
- Purpose:
- To store all the information of a single room within the salon and the pets within the room
- Fields:
char room_name[MAX_NAME_LEN]
- The name of the room
enum pet_type pet_type
- The type of pet as an enum
- Can be either
CAT
,DOG
,RABBIT
orPARROT
int num_pets
- Number of pets to be cared for in a room
struct pet_room *next
- The next room in the pet salon
struct financial_summary
- Purpose:
- To store all the financial information of the salon
- Fields:
int total_cared
- The number of pets that have been cared for
double total_profit
- Total profit from the number of pets being cared for
Additionally, you can create your own enums if you would like, but you should not modify the provided pet_type
enum.
Reference Implementation
To help you understand the proper behaviour of the CS Pet Salon, we have provided a reference implementation. If you have any questions about the behaviour of your assignment, you can check and compare it to the reference implementation.
To run the reference implementation, use the following command:
1091 cs_pet_salon
How to get started
There are a few steps to getting started with CS Pet Salon.
- Create a new folder for your assignment work and move into it.
mkdir ass2 cd ass2
Download cs_pet_salon.c here
Or, copy these file(s) to your CSE account using the following command:
1091 fetch-activity cs_pet_salon
Run
1091 autotest cs_pet_salon
to make sure you have correctly downloaded the file.
1091 autotest cs_pet_salon
- Read through Stage 1.
About the starter code
The starter code contains some provided functions to help simplify some stages of the assignment. These functions have been fully implemented for you and should not need to be modified to complete the assignment.
These provided functions will be explained in the relevant stages of the assignment.
Please read the comments and the assignment specification as we will suggest certain provided functions for you to use.
It also contains two function stubs, create_salon
and create_room
, which you will need to complete in Stage 1.1.
Finally, the main
function contains some comments to help guide you through Stage 1.1, as well as some printf
messages which run when the program starts and ends.
These printf
messages are:
Welcome to 1091 CS Pet Salon manager! =^.^= All pet salons closed! =^.^=
Allowed C Features
In this assignment, you cannot use arrays, other than char
arrays for strings, and cannot use the features explicitly banned in the Style Guide.
We strongly encourage you to complete the assessment using only features taught in lectures up to and including weeks 8 and 9. The only C features you will need to get full marks in the assignment are:
int
,char
, anddouble
variables.- Enums.
- Structs.
- If statements.
- While and for loops.
- Your own functions.
- Pointers.
char
arrays/strings (you are not allowed to use arrays that are notchar
arrays).- Linked lists.
- Good Code Style!
(Header comments, function comments, constants (#define
's), and whitespace and indentation.)
Using any other features will not increase your marks (and will make it more likely you make style mistakes that cost you marks).
If you choose to disregard this advice, you must still follow the Style Guide. You also may be unable to get help from course staff if you use features not taught in (DPST1091|ADMN0112).
Features that the Style Guide labeled as illegal will be penalized during marking.
FAQ
Your Tasks
This assignment consists of four stages. Each stage builds on the work of the previous stage, and each stage has a higher complexity than its predecessor. You should complete the stages in order.
Stage 1
For Stage 1 of this assignment, you will be implementing the command loop, as well as the commands to add pet rooms to a pet salon.
Specifically, this will include:
- Implementing the
create_salon
andcreate_room
functions. - Implementing the command loop, to scan commands until
CTRL-D
. - Adding rooms to the end of the salon.
- Printing out all rooms in the salon.
- Handling errors.
- Inserting adjacent room.
By the end of this stage, your linked list of pet rooms will look something like:
Stage 1.1 - Creating a salon and room
As you might have found by now, it can be really useful to have a function that takes the input for a linked list node, calls malloc
and initialises all the fields of the node. So, in Stage 1.1, we will be implementing functions that do exactly that for a struct salon
, and for a struct pet_room
.
You'll find the following unimplemented functions in the starter code:
// TODO: what does this function do?
//
// Paramters:
// TODO: explain what your parameters are here!
// Returns:
// TODO: explain what your function returns here!
struct salon *create_salon(char salon_name[MAX_NAME_LEN], double base_cost) {
// STAGE 1.1
// TODO: malloc, initialise, and return a new salon.
// hint: you will have to replace NULL in this return statement.
return NULL;
}
// TODO: what does this function do?
//
// Paramters:
// TODO: explain what your parameters are here!
// Returns:
// TODO: explain what your function returns here!
struct pet_room *create_room(char room_name[MAX_NAME_LEN], enum pet_type pet_type) {
// STAGE 1.1
// TODO: malloc, initialise, and return a new room.
// hint: you will have to replace NULL in this return statement.
return NULL;
}
Your task is to complete the create_salon
function, so that it:
- Malloc's a new
struct salon
. - Copies the
salon_name
andbase_cost
into the corresponding struct field. - Initialise the nested
struct financial_summary
such thattotal_cared
andtotal_profit
are zero. - Assign
next
androoms
toNULL
. - Returns a pointer to the malloc'd struct.
Your also then need to complete the create_room
function, so that it:
- Malloc's a new
struct pet_room
. - Copies the
room_name
andpet_type
into the corresponding struct fields. - Initialises all other fields to some either zero equivalent or
NULL
. - Returns a pointer to the malloc'd struct.
Error Conditions
- There is no error handling required for this stage. You'll be adding this later in Stage 1.4 - Handle Errors.
Testing
There are no autotests for Stage 1.1.
Instead, you may want to double check your work by compiling your code using dcc
and making sure there are no warnings or errors. If you manually tested ./cs_pet_salon
, it would only print the lines in main.
As you can tell, this does not test the functions you just implemented. You could also write some temporary testing code to check your create_salon
and create_room
functions work properly.
For example, you could copy the following testing code into your main function:
///////////////////////////// TESTING CODE /////////////////////////////
// name of pet room
char name[MAX_NAME_LEN] = "blue";
// create a struct room with
// room_name : "blue"
// pet_type : CAT
struct pet_room *test_room = create_room(name, CAT);
// print out all of its fields.
printf("room name: %s\n", test_room->room_name);
printf("number of pets: %d\n", test_room->num_pets);
if (test_room->pet_type == CAT) {
printf("pet type: cat\n");
} else {
printf("pet type: not a cat\n");
}
if (test_room->next == NULL) {
printf("next field: NULL");
}
///////////////////////////// TESTING CODE /////////////////////////////
This code just calls create_room
to malloc and initialise a struct pet_room
, and then prints out all of its fields.
If you run it, it should print out something like:
room name: blue number of pets: 0 pet type: cat next field: NULL
Stage 1.2 - Add room
Now we'll be implementing the command loop, allowing your program to take in and perform different operations on the salon.
From this stage onwards, your program should run in a loop, scanning in and executing commands until CTRL-D
. You should implement this command loop between the existing welcome and goodbye messages in the starter code.
On each iteration of the loop, your program should:
- Print the prompt
Enter command:
. - Scan in a command character.
- Scan in any arguments following the command character and execute the command.
Each command will start with a single unique character, and may be followed by a variable number of arguments depending on the command.
The unique character for each different command and the number and type of the command arguments are specified in the relevant stages of this assignment.
The first command you have to implement is the ADD ROOMS command, which is described below.
When you run your program, a new salon should be created in main with the name "cs_salon"
and base cost of 10.2
. The name has been already given to you in main with the variable salon_name
so you can pass it through the create_salon
function.
This salon will start out empty - with no rooms.
It should look something like this:
To make your salon more useful, we need a way of adding a room to the end of the list of rooms.
Command: Add room
a [room_name] [pet_type]
Description
The a
command takes in 2 arguments:
- a string called
room_name
, - an
enum pet_type
calledpet_type
.
Some helper functions have been provided to help you scan in these arguments:
void scan_name(char string[MAX_NAME_LEN])
enum pet_type scan_pet_type()
You can find more information on these here: Provided helper functions.
When the a
command is entered, your program should create a new room containing the room_name
, pet_type
, and num_pets
, then append it to the end of the list of rooms inside the salon (in other words, insert the new room at the end of the salon's rooms
linked list).
Finally, it should out a message to confirm the command was successful:
"Room: '[room_name]' added!\n"
You should replace [room_name]
with the room_name
you scanned in.
For example, if we have just started the program and we use the a
command once, it would look like:
Welcome to 1091 CS Pet Salon manager! =^.^= Enter command: a blue cat Room: 'blue' added!
Our linked list should now look like this:
If we then run the a
command again:
Enter command: a happy dog Room: 'happy' added!
then our linked list should now look like:
Provided helper functions
Two helper functions have been provided for this stage: void scan_name(char string[MAX_NAME_LEN])
:
char string[MAX_NAME_LEN]
: used to scan theroom_name
.
enum pet_type scan_pet_type()
:
- Scans the
pet_type
and returns it as anenum pet_type
. - Returns
INVALID_PET_TYPE
if thepet_type
did not correspond to one of the valid pet types.
You should use these functions to help you scan in the room_name
and pet_type
arguments.
Remember that the arguments must be scanned in the correct order, so your code to scan arguments will look something like the following:
// Create variables to scan arguments into
char room_name[MAX_NAME_LEN];
enum pet_type type;
// Arguments are in order: [room_name] [pet_type]
// 1. Scan room_name first
scan_name(room_name);
// 2. Then scan the pet_type
type = scan_pet_type();
// We've scanned in all the arguments! Now we can use them in our code
Error Conditions
- There is no error handling required for this stage. You'll be adding this later in Stage 1.4 - Handle Errors.
Assumptions
salon_name
will always be less thanMAX_NAME_LEN
including their null terminator at the end.salon_name
will not contain any whitespace. For examplemy_salon
is a valid title butmy salon
is not. We will not test any invalidsalon_name
orroom_name
inputs so you do not need to account for this in your program.salon_name
will not contain any quotations. For examplebobs
is a valid title butbob's
is not. We will not test any invalidsalon_name
orroom_name
inputs so you do not need to account for this in your program.- All input will be in lowercase. Uppercase characters will not be tested.
pet_type
will be entered as a lowercase string and automatically converted to the correctenum pet_type
for you by thescan_pet_type
function, when the function is used.
Corresponding string | enum pet_type |
---|---|
"cat" | CAT |
"dog" | DOG |
"rabbit" | RABBIT |
"parrot" | PARROT |
any invalid string | INVALID_PET_TYPE |
Note that you don't need to worry about INVALID_PET_TYPE
until Stage 1.4.
Until then, you can assume that the returned enum pet_type
will never be INVALID_PET_TYPE
.
Examples
Stage 1.3 - Printing rooms in the salon
Now we want a way to display the salon and all its rooms.
Command: Print rooms
p
Description
The p
command takes no arguments.
When the p
command is run, your program should print out all rooms in the current salon, from head to tail.
The print_one_room
function has been provided for you to format and print a single room. More information on its usage can be found below. After all the rooms are printed, the following line should be printed:
All the rooms listed above are in salon '[salon_name]'.
where [salon_name]
is the name of salon containing the rooms being printed.
If there are no rooms in the salon, you should print the following message instead:
There are no pet rooms in this salon!
Provided helper functions
One helper function has been provided for this stage:
void print_one_room(int position, struct pet_room *room)
int position
: the position of where the room is in the salon. (In other words, if this room was the first in the linked list, the position would be 1).struct pet_room *room
: a pointer to a pet room.
This function will print out the required information for the pet room in the correct format. Your job is to determine the correct values to pass to this function.
This means you don't need to worry about copying the exact output format for the p
command. To match the autotests exactly, you should loop through the list of pet rooms and call this function for each of them.
Error Conditions
- There is no error handling required for this stage. You'll be adding this later in Stage 1.4 - Handle Errors.
Examples
Stage 1.4 - Handle Errors
Once you've reached this stage, you should be able to add rooms to your salon and print them! Nice work!
However, the people using your salon system can still make mistakes! Let's consider this example:
Welcome to 1091 CS Pet Salon manager! =^.^= Enter command: a blue cat Room: 'blue' added! Enter command: a blue cat Room: 'blue' added! Enter command: p /-------------------------------\ Room name: blue Room position: 1 Pet type: cat Num of pets in room: 0/10 \-------------- | --------------/ V /-------------------------------\ Room name: blue Room position: 2 Pet type: cat Num of pets in room: 0/10 \-------------- | --------------/ V All the rooms listed above are in salon 'cs_salon'. Enter command: All pet salons closed! =^.^=
It does not make sense to have two rooms with the same name - this can be confusing for the owner! We need to ensure that the room names are unique. Also, there are only a few types of pets that the salon can look after, so we want to make sure that the input for the type of pet is actually valid for the salon.
In this stage you will be modifying your code from Stage 1.3 so we make sure only valid rooms can be added to your salon.
Error Conditions
When running the a
command, you scanned in the room_name
and pet_type
for the new room.
If any one of the following conditions are met, then you should not append a new room to the linked list. You should instead print out an error message:
- If there is already a room in the linked list that contains the same
room_name
, the following error should be printed:Error: This room name already exists!
- If
pet_type
(returned by thescan_pet_type
function) isINVALID_PET_TYPE
, the following error should be printed:Error: Unfortunately, this salon does not cater for this pet type!
Using the strcmp
function in string.h
might be useful for this step!
Clarifications
- If more than one error occurs, only the first error should be addressed by printing an error message. For example, if there is already a room name that exists and the pet type does not exist - only the room name error should be printed out.
- As before, you can assume there is no whitespace within a
room_name
and do not need to do any error handling for this. - As before, uppercase characters are not being tested.
- This is the same for all future commands with error checking.
Examples
Stage 1.5 - Insert adjacent room
We want to make sure all pets are comfortable at their stay at the salon. We noticed that putting some pets adjacent to pets of the same type helps with this! For example when making a new room and we already see that there's a room existing for cats, we want to place this new room next to that existing room. This is similar to Stage 1.2 - Add room, however position matters for this command.
Command: Insert adjacent room
i [room_name] [pet_type]
Description
The i
command is similar to the a
command, except the position of the room matters.
It should create a new struct pet_room
containing room_name
, pet_type
and num_pets
. If a room exists of the same pet_type
, then the new room should be inserted after that existing room. If the same pet_type is not found, then the room should be inserted at the end.
For example if we ran the following commands:
a blue cat
a happy parrot
resulting in the following.
Then used our new command:
i quiet cat
the room would be inserted after the same pet_type is found as seen below.
After successful insertion, your program should print the following message:
Room: '[room_name]' inserted!
Provided helper functions
See Stage 1.2 - Add room for information on how to scan the room_name
and pet_type
.
Error Conditions
Just like command a
: add room, there are restrictions on the rooms that can be inserted into the salon.
Specifically, if any of the previous mentioned error conditions are met, then the room should not be inserted, and an error message should be printed out instead. Please refer to Error Conditions in Stage 1.4 - Handle Errors for more information.
Examples
Testing and Submission
Remember to do your own testing
Are you finished with this stage? If so, you should make sure to do the following:
- Run
1091 style
, and clean up any issues a human may have reading your code. Don't forget -- 20% of your mark in the assignment is based on style and readability! - Autotest for this stage of the assignment by running the
autotest-stage
command as shown below. - Remember -- give early, and give often. Only your last submission counts, but why not be safe and submit right now?
1091 style cs_pet_salon.c 1091 autotest-stage 01 cs_pet_salon give dp1091 ass2_cs_pet_salon cs_pet_salon.c