COMP1511 Programming Fundamentals - Assignment 2 - CS Dungeon!
Assignment 2 - CS Dungeon!
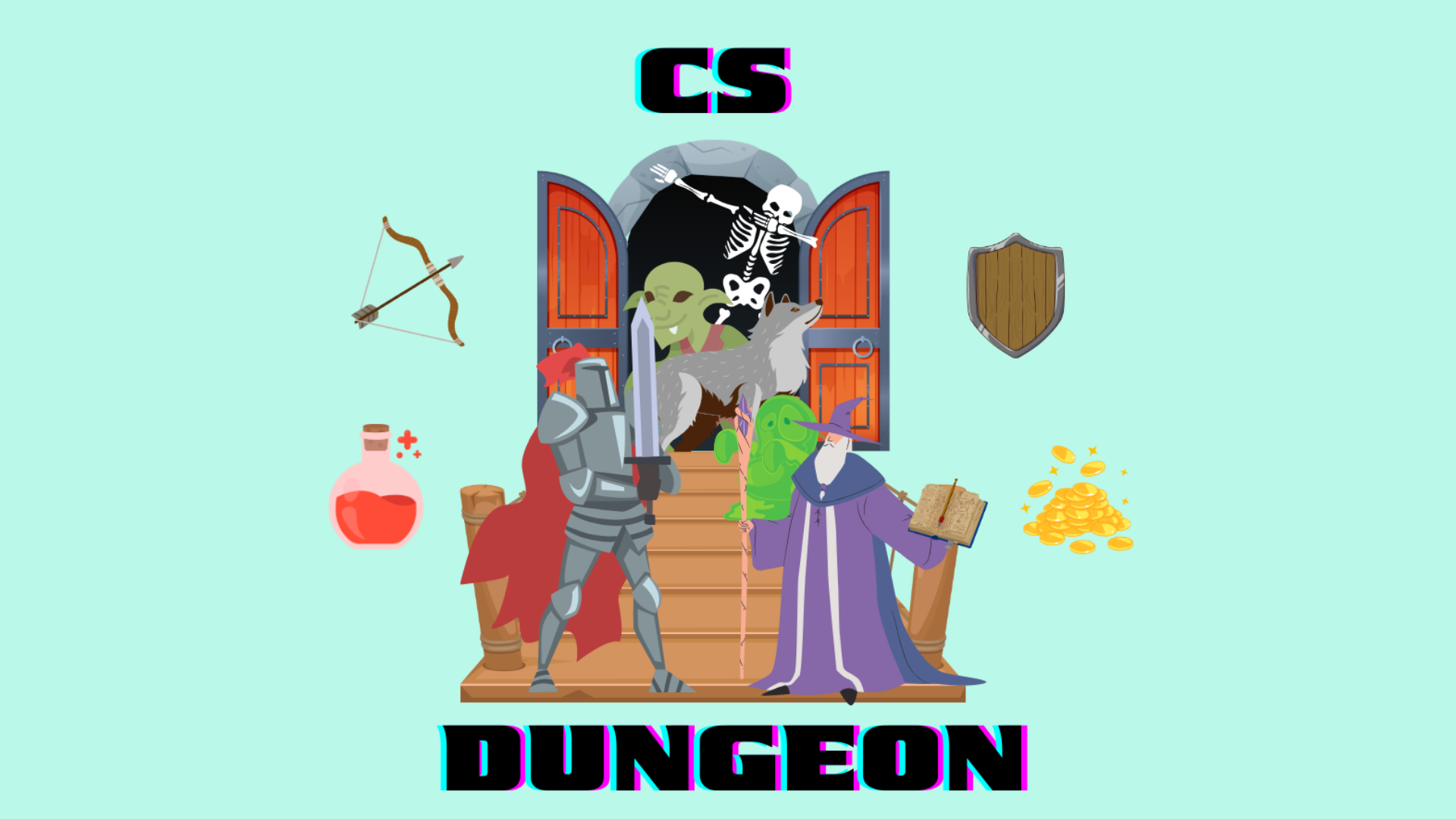
The game consists of a setup phase, where you add dungeons and items, and a gameplay phase, where you as the player will get to move between dungeons, fight monsters and collect items that can make you even stronger!
You can read about the history of dungeon crawler games here.
Overview
COMP1911 Students
If you are a COMP1911 student, you will be assessed on your performance in stages 1 and 2 ONLY for this assignment, which will make up the performance component of your grade. Style will be marked as usual and will make up the remaining 20% of the marks for this assignment. You are NOT required to attempt stages 3 and 4. You may attempt the later stages if you wish, but you will not be awarded any marks for work beyond stage 2.
COMP1511 Students
If you are a COMP1511 student, you will be assessed on your performance in stages 1 through 4, which will make up the performance component of your grade. Style will be marked as usual and will make up the remaining 20% of the marks for this assignment.
Assignment Structure
This assignment will test your ability to create, use, manipulate and solve problems using linked lists. To do this, you will be implementing a dungeon crawler game, where the dungeons are represented as a linked list, stored within a map. Each dungeon contains a list of items. The map also contains a player struct, which also contains a list of items.
We have defined some structs in the provided code to get you started. You may add fields to any of the structs if you wish.
The following enum definitions are also provided for you. You can create your own enums if you would like, but you should not modify the provided enums.
Getting Started
There are a few steps to getting started with CS Dungeon.
- Create a new folder for your assignment work and move into it. You can follow the commands below to link and copy the files.
mkdir ass2 cd ass2
- There are 3 files in this assignment. Run the following command below to download all 3, which will link the header file and the main file. This means that if we make any changes to
cs_dungeon.h
ormain.c
, you will not have to download the latest version as yours will already be linked.
1511 fetch-activity cs_dungeon
- Run
1511 autotest cs_dungeon
to make sure you have correctly downloaded the file.
1511 autotest cs_dungeon
- Read through the rest of the introductory specification and Stage 1.
Starter Code
This assignment utilises a multi-file system. There are three files we use in CS Dungeon:
Main File (
main.c
): This file handles all input scanning and error handling for you. It also tests your code incs_dungeon.c
and contains themain
function. You don't need to modify or fully understand this file, but if you're curious about how this assignment works, feel free to take a look. You cannot changemain.c
.Header File (
cs_dungeon.h
): contains defined constants that you can use and function prototypes. It also contains header comments that explain what functions should do and their inputs and outputs. If you are confused about what a function should do, read the header file and the corresponding specification. You cannot changecs_dungeon.h
.Implementation File (
cs_dungeon.c
): contains stubs of functions for you to implement. This file does not contain amain
function, so you will need to compile it alongsidemain.c
. This is the only file you may change. You do not need to usescanf
orfgets
anywhere.
The implementation file cs_dungeon.c
contains some provided functions to help simplify some stages of this assignment. These functions have been fully implemented for you and should not need to be modified to complete this assignment.
These provided functions will be explained in the relevant stages of this assignment. Please read the function comments and the specification as we will suggest certain provided functions for you to use.
How to Compile CS Dungeon
Reference Implementation
To help you understand the expected behaviour of CS Dungeon, we have provided a reference implementation. If you have any questions about the behaviour of your assignment, you can check and compare yours to the reference implementation.
To run the reference implementation, use the following command:
1511 cs_dungeon
The easiest way to understand how this assignment works is to play a game yourself! Below is example input you can try by using the reference solution.
Allowed C Features
In this assignment, there are no restrictions on C Features, except for those in the Style Guide. If you choose to disregard this advice, you must still follow the Style Guide.
You also may be unable to get help from course staff if you use features not taught in COMP1511. Features that the Style Guide identifies as illegal will result in a penalty during marking. You can find the style marking rubric above. Please note that this assignment must be completed using only Linked Lists . Do not use arrays in this assignment. If you use arrays instead of linked lists you will receive a 0 for performance for this assignment.
Banned C Features
In this assignment, you cannot use arrays for the list of dungeons nor the lists of items and cannot use the features explicitly banned in the Style Guide. If you use arrays in this assignment for the linked list of dungeons or the linked lists of items you will receive a 0 for performance in this assignment.
FAQ
Game Structure
This game consists of a setup phase and a gameplay phase.
You will be implementing both the setup phase and gameplay phase throughout this assignment, adding more features to both as you progress. By the end of **stage 1.4****you will have implemented parts of both setup and gameplay enough to play a very basic game.
The game is ended either by the user entering Ctrl-D
, the win condition being met, or the player running out of health points. The program can also be ended in the setup phase with Ctrl-D
or q
.
Your Tasks
This assignment consists of four stages. Each stage builds on the work of the previous stage, and each stage has a higher complexity than its predecessor. You should complete the stages in order.
A video explanation to help you get started with the assignment can here found here:
Stage 4
This stage is for students who want to challenge themselves, and solve more complicated linked lists and programming problems, such as:
- Teleportation between dungeons
- Defeating the final boss
Stage 4.1 - Teleportation Between Dungeons
In Stage 4.1, we’d like to be able to teleport the player between dungeons.
Enter Command: T
All dungeons can be teleported to. When the T
command is entered, the player should teleport to the furthest dungeon from the current dungeon they are in, unless that dungeon has already been teleported to. Once all dungeons have been teleported to, the pattern can begin again.
For example, a map with 4 dungeons. If the player starts in the first dungeon, their movements would be as follows (the player is represented by the knight character):
There is one function in cs_dungeon.c
that you will have to implement for Stage 4.1, teleport
. INVALID
should be returned when there is only one dungeon in the map, otherwise, VALID
should be returned.
Clarifications
- Whenever the map is changed (by shuffling in Stage 4.2 or by removing empty dungeons), the teleportation movement should restart, as if no dungeons have been teleported to yet.
- When the player moves between dungeons with
>
or<
, the teleportation movement should restart, as if no dungeons have been teleported to yet. - If multiple dungeons are equidistant, the player should travel to the first one in the map.
- The first dungeon the player starts the teleportation cycle in counts as being teleported to.
Examples
Stage 4.2 - The Final Boss
We have finally reached the final boss! When the final boss is attacked for the first time, it becomes so enraged, spreading its power across the dungeon, shaking things up so that the map becomes unrecognisable. In this stage you will be implementing the boss_fight
function.
Enter Command: b
To be able to attack the final boss, the player must have the required item type in their inventory, not yet used.
When the player attacks the final boss, they should deal either physical damage or magical damage, as calculated in fight
from Stage 2.4. They should do whichever does more damage. If the damage is equal, they should deal magical damage.
The first time the boss is attacked, the map should be shuffled, so that pairs of dungeons switch places. Below is an example diagram of a dungeon map containing 4 dungeons:
When attacking a boss, the boss can sustain damage, and can be damaged across multiple turns. Bosses will fight back each turn the player is in the same dungeon, and when they reach 50% of their original health or less, they will do 1.5 times more damage.
Bosses will follow the player (but cannot teleport), and will attempt to reach the player by moving towards them each turn. If they are in the same dungeon as the player, they will attack. Bosses can move or attack, but not both in the same turn.
You will need to add code to your end_turn
function. A turn should run as follows:
- Player action (including shuffling the map if it is the first time the boss is attacked)
- Monster attacks
- Remove any empty dungeons
- Boss attack or move
- Check if the game is over
If the player defeats the boss, then you should call the helper function print_boss_defeat
after both the player and the boss have done their actions for the turn.
Clarifications
- If there is an odd number of dungeons, e.g. 5 dungeons, swap the first 4, leaving the last dungeon in its original position.
boss_fight
should returnNO_BOSS
when there is no boss present in the current dungeon,NO_ITEM
when the player does not have the required item to fight the boss, andVALID
otherwise.- Make sure to go back and check your code from Stage 3.1 to check if the boss has been defeated. If the boss is defeated, their points should be added to the player's point total.
- Whenever the map is changed, the teleportation movement Stage 4.1 should restart, as if no dungeons have been teleported to yet.
- The boss only start moving once attacked for the first time.
- You may assume the same shield power logic as Stage 2.4 applies to the boss damage.
Examples
Testing and Submission
Remember to do your own testing
Are you finished with this stage? If so, you should make sure to do the following:
- Run
1511 style
and clean up any issues a human may have reading your code. Don't forget -- 20% of your mark in the assignment is based on style and readability! - Autotest for this stage of the assignment by running the
autotest-stage
command as shown below. - Remember -- give early and give often. Only your last submission counts, but why not be safe and submit right now?
1511 style cs_dungeon.c 1511 autotest-stage 04 cs_dungeon give cs1511 ass2_cs_dungeon cs_dungeon.c